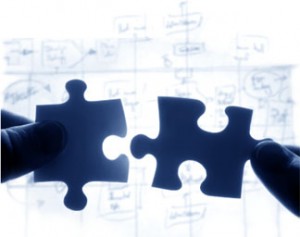
Parsing Data
As part of rebuilding my website from scratch, I was longing to display the titles (and links) of my recent blog articles. There are many RSS fetcher implementations online but I wanted to make one by myself (Breath of fresh air 😛 ).
Initially, all I could think of is using a Web Service to bring in the data using SOAP and then ripping through it to fetch the titles and links. But there are nifty tools as part of C# which we can use to get the same data. I zeroed in the WebClient class which is available as part of System.NET API and we just have to pass in the URI and get a steady stream of data :).
Remaining work revolves around parsing/chopping/filtering/nunchuck”-ing” through the downloaded data! \o/
Next, we use efficient data structures to hold the parsed data, flatten the data into a string variable and then call the variable by using <% =STRING_VARIABLE_NAME%> inside an asp.net page and display it the way we want. I displayed it inside a simple marquee tag at present and will be extending it further soon.
Source Code >> BlogData.cs
>>>>Updated copy of the above source code which does not include management of the intermediate temp file >> BlogData2.cs
(Replace the “.cs_.txt” extension to “.cs” before using the file)
{
public string title;
public string link;
}
class BlogData
{
public string GetData()
{
//
// TODO: Add constructor logic here
//
String finalString = string.Empty;
List<String> titles = new List<string>();
List<String> links = new List<string>();
List<BogEntity> entityList = new List<BogEntity>();
using (WebClient client = new WebClient())
{
using (Stream myStream = client.OpenRead(“http://gauravpandey.com/wordpress/?feed=rss2″))
{
using (StreamReader reader = new StreamReader(myStream))
{
StringBuilder sb = new StringBuilder();
String data = string.Empty;
while ((data = reader.ReadLine()) != null)
sb.Append(data+”\n”);
finalString = sb.ToString();
}
}
}
using (StreamWriter writer = new StreamWriter(HttpContext.Current.Server.MapPath(“temp.txt”)))
{
writer.WriteLine(finalString);
}
finalString = string.Empty;
string defaultURL = “http://gauravpandey.com/wordpress/?p=”;
using (StreamReader reader = new StreamReader(HttpContext.Current.Server.MapPath(“temp.txt”)))
{
string line = string.Empty;
while((line = reader.ReadLine()) != null)
{
if(line.Contains(“<title>”) && !line.Contains(“Weblog”))
{
titles.Add(line.Replace(“<title>”, string.Empty).Replace(“</title>”, string.Empty).Trim());
}
else if (line.Contains(defaultURL) && line.Contains(“<link>”) && line.Contains(“</link>”))
{
links.Add(line.Replace(“<link>”, string.Empty).Replace(“</link>”, string.Empty).Trim());
}
else
{
//all the remaining garbage data
}
}
}
int len = titles.Count;
for(int i = 0; i < len; i++)
{
BogEntity objX = new BogEntity();
objX.title = titles[i];
objX.link = links[i];
if (!objX.title.Equals(“GauZ's Weblog”) && !objX.link.Equals(“http://gauravpandey.com/wordpress”))
entityList.Add(objX);
}
titles.Clear();
titles = null;
links.Clear();
links = null;
StringBuilder sbX = new StringBuilder();
sbX.Append(“Recent blog articles by Gaurav from WordPress >>Â “);
foreach(BogEntity x in entityList)
{
sbX.Append(“<a href=\”” + x.link + “\” target=\”_blank\”>” + x.title + “</” + “a> “);
}
return sbX.ToString();
}
}
Login